Arduino release several new boards including the Nano 33 BLE which pick my interest due to the fact that it is based on the Nrf52480 chip ( https://www.nordicsemi.com/Products/Low-power-short-range-wireless/nRF52840) which should be compatible with Bluetoot MESH. I decided to give it a try…
This is the first board based on the Nrf52840 and thus there is no existing Arduino core for this board which means they would face some challenge to have Arduino running on it. They went for a smart solution by deciding to run Arduino Core on top of MBED OS to be able to use all the works already done by MBEDOS to integrate the nrf52840 chip. This is explain on their blog if you are curious: https://blog.arduino.cc/2019/07/31/why-we-chose-to-build-the-arduino-nano-33-ble-core-on-mbed-os/
That’s when I discover about MBEDOs ” Arm Mbed OS is a free, open-source embedded operating system designed specifically for the “things” in the Internet of Things. ” https://www.mbed.com/en/platform/mbed-os/ which seems promising especialy since it has a very complet Bluetooth stack Cordio (https://os.mbed.com/docs/mbed-cordio/19.02/introduction/index.html) which include Bluetooth MESH capability (https://community.arm.com/iot/b/blog/posts/bluetooth-mesh-with-bluetooth-5-and-cordio-radio-ip) which is not offer today by the Arduino Bluetooth library (https://github.com/arduino-libraries/ArduinoBLE/issues/22). That’s why i decided to give a try to program with MBEDos directly instead of Arduino. Nevertheless my first idea was to keep the arduino bootloader on the board so that I can program it WITHOUT any extra device and benefits from arduino bootloader.
Code
I used MBED studio to avoid dealing with the setup of a toolchain on windows: https://os.mbed.com/studio/
The only “difficulty” is to use a mbed version recent enough (>=5.14) to have the adruino nano 33 in the list of target.
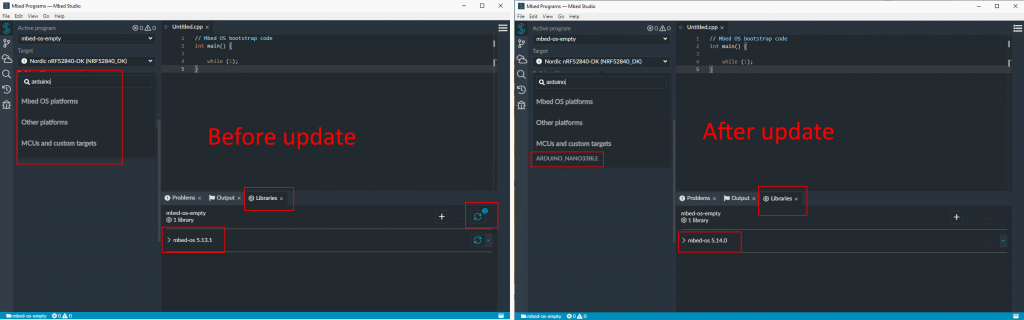
I ve done a very simple program that will blink 3 times a LED on pin
#include "mbed.h" #include "ble/BLE.h" DigitalOut led1(p47); // main() runs in its own thread in the OS int main() { //Pattern is 3 blink and wait while (true) { // Turn led on led1 = 1; wait_ms(200); led1 = 0; wait_ms(200); led1 = 1; wait_ms(200); led1 = 0; wait_ms(200); led1 = 1; wait_ms(200); led1 = 0; wait_ms(3000); } }
Also in the folder of your project you should have a /mbed-os/targets/targets.json with the NANO33 definition.
I added 2 parameters “”OUTPUT_EXT”: “bin”” to generate a bin file instead of an hex file (the uploader use bin files)
The second change is “”mbed_app_start”: “0x10000″” so that the toolchain know my program will be located in memory at address 0x10000. This info is part of the arduino bootlaoder code and was explain to me here: https://github.com/arduino/ArduinoCore-nRF528x-mbedos/issues/19 The arduino bootloader will call the code at this address after it boot.
After you compile the code with MBED studio you will have the result binary here “C:\Users\charl\Mbed Programs\mbed-os-example-blinky\BUILD\ARDUINO_NANO33BLE\ARMC6” that can be uploaded to the board
upload
This part is tricky since my original goal is to use the arduino bootloader to upload my code to the board without any other tools. It took me some time to understand how to do it but hopefuly i got some help here https://github.com/arduino/ArduinoCore-nRF528x-mbedos/issues/19 and so i decided to use BOSSAC which is the same tools used by the arduino IDE. You can see it in the logs if you activate the extra logs in the IDE.
I re-use the bossac binary that is shipped with the arduino IDE and added an option to upload at address 0x10000. You need to press twice the reset button on the board to force the bootloader to run forever before starting the upload command.
C:\Users\charl\AppData\Local\Arduino15\packages\arduino\tools\bossac\1.9.1-arduino1> .\bossac.exe -d --port=COM5 -o 0x10000 -U -i -e -w 'C:\Users\charl\Mbed Programs\mbed-os-example-blinky\BUILD\ARDUINO_NANO33BLE\ARMC6\mbed-os-example-blinky.bin' -R Set binary mode version()=Arduino Bootloader (SAM-BA extended) 2.0 [Arduino:IKXYZ] Connected at 921600 baud identifyChip()=nRF52840-QIAA write(addr=0,size=0x34) writeWord(addr=0x30,value=0x400) writeWord(addr=0x20,value=0) version()=Arduino Bootloader (SAM-BA extended) 2.0 [Arduino:IKXYZ] Device : nRF52840-QIAA Version : Arduino Bootloader (SAM-BA extended) 2.0 [Arduino:IKXYZ] Address : 0x0 Pages : 256 Page Size : 4096 bytes Total Size : 1024KB Planes : 1 Lock Regions : 0 Locked : none Security : false Erase flash chipErase(addr=0x10000) Done in 0.003 seconds Write 43884 bytes to flash (11 pages) [ ] 0% (0/11 pages)write(addr=0x34,size=0x1000) writeBuffer(scr_addr=0x34, dst_addr=0x10000, size=0x1000) [== ] 9% (1/11 pages)write(addr=0x34,size=0x1000) writeBuffer(scr_addr=0x34, dst_addr=0x11000, size=0x1000) [===== ] 18% (2/11 pages)write(addr=0x34,size=0x1000) writeBuffer(scr_addr=0x34, dst_addr=0x12000, size=0x1000) [======== ] 27% (3/11 pages)write(addr=0x34,size=0x1000) writeBuffer(scr_addr=0x34, dst_addr=0x13000, size=0x1000) [========== ] 36% (4/11 pages)write(addr=0x34,size=0x1000) writeBuffer(scr_addr=0x34, dst_addr=0x14000, size=0x1000) [============= ] 45% (5/11 pages)write(addr=0x34,size=0x1000) writeBuffer(scr_addr=0x34, dst_addr=0x15000, size=0x1000) [================ ] 54% (6/11 pages)write(addr=0x34,size=0x1000) writeBuffer(scr_addr=0x34, dst_addr=0x16000, size=0x1000) [=================== ] 63% (7/11 pages)write(addr=0x34,size=0x1000) writeBuffer(scr_addr=0x34, dst_addr=0x17000, size=0x1000) [===================== ] 72% (8/11 pages)write(addr=0x34,size=0x1000) writeBuffer(scr_addr=0x34, dst_addr=0x18000, size=0x1000) [======================== ] 81% (9/11 pages)write(addr=0x34,size=0x1000) writeBuffer(scr_addr=0x34, dst_addr=0x19000, size=0x1000) [=========================== ] 90% (10/11 pages)write(addr=0x34,size=0x1000) writeBuffer(scr_addr=0x34, dst_addr=0x1a000, size=0x1000) [==============================] 100% (11/11 pages) Done in 2.076 seconds reset() PS C:\Users\charl\AppData\Local\Arduino15\packages\arduino\tools\bossac\1.9.1-arduino1>
Seems to be working… nevertheless the LED do not blink 🙁
In the meantime I bought a programmer for the chip since the manufacturer has a “education/hobbyist” version for only 20$… https://www.segger.com/products/debug-probes/j-link/models/j-link-edu-mini/ I was not aware that i could had a official programmer for such low price….which made my original idea to reuse the Arduino bootloader to upload to avoid buying one useless…
Now that I have my hand on a programmer i decided to try to understand why my program was not running….is it my code or the upload failed or something else. I modify my code to remove the offset and start at address 0x0 and I upload it directly on the chip and the LED start blinking. The code is thus good and the issue seems located in the upload process… I decided to do another test and put back my offset 0x10000 in my program, erase the board, upload the Arduino bootloader in the board, and finally upload my program at address 0x10000 but not with BOSSAC but using the J-Link tool. The LED start blinking !
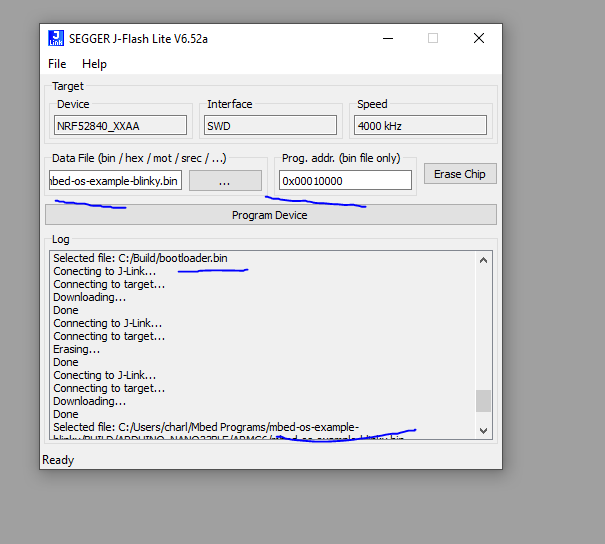
I compare the memory at address 0x10000 for both cases. First the test where i upload with BOSSAC at address 0x10000 and my program do not works
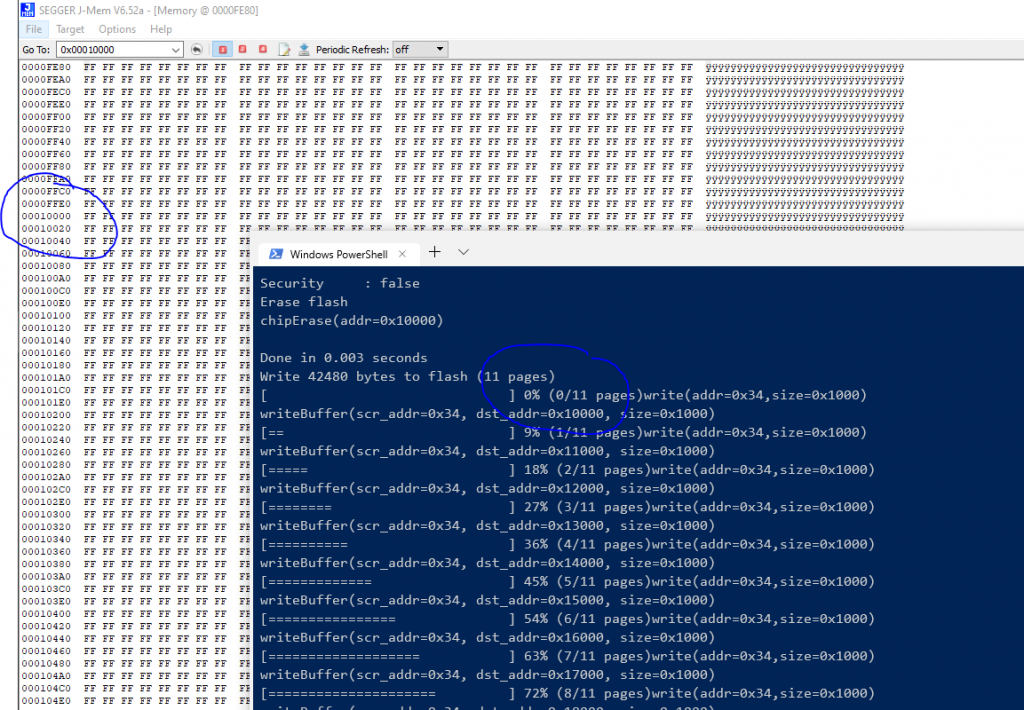
and when I upload with J-Link
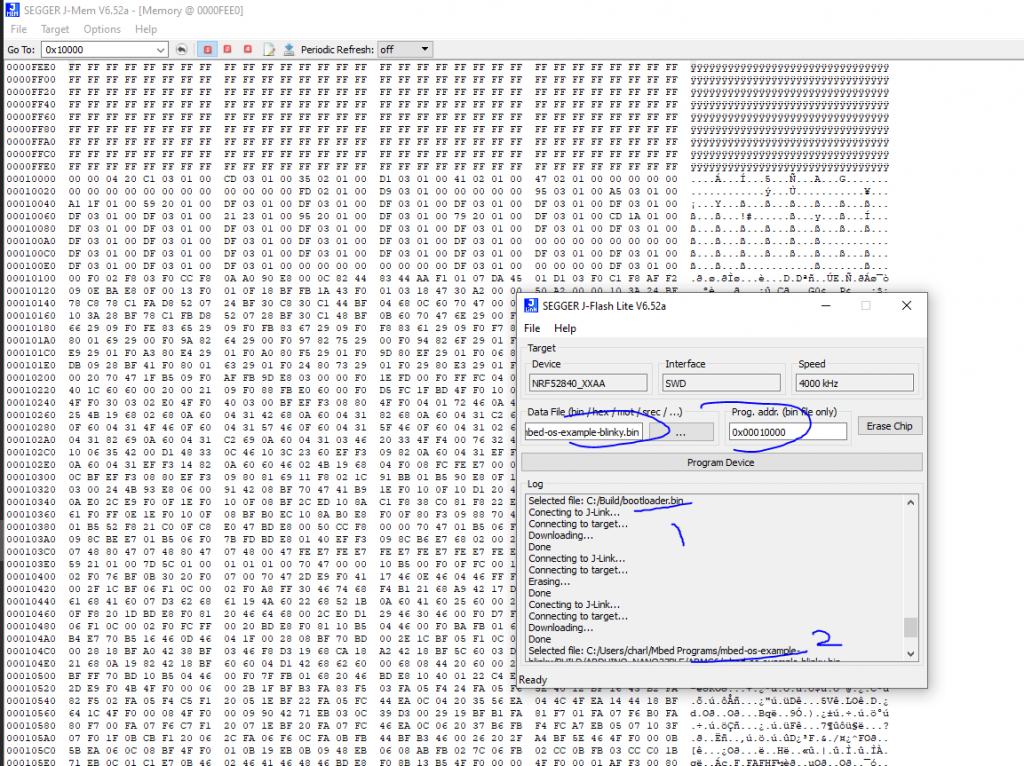
It seems the upload with BOSSAC do not works which seems to confirms what i thought.
Conclusion
I stop here since I now have a programmer to directly program the chip and so I can move on with my Bluetooth MESH tests.
Thx to https://github.com/facchinm for helping me understand more about Arduino bootloader and Thx to https://www.segger.com/ for selling a powerful programmer for hobbyist at a very reasonable price.
If you want to try MBED OS you should take a board compatible offering a DAP-Link for USB upload “Arm Mbed DAPLink is an open-source software project that enables programming and debugging application software on running on Arm Cortex CPUs. Commonly referred to as interface firmware, DAPLink runs on a secondary MCU that is attached to the SWD or JTAG port of the application MCU. This configuration is found on nearly all development boards. It creates a bridge between your development computer and the CPU debug access port. DAPLink enables developers with drag-and-drop programming, a serial port and CMSIS-DAP based debugging.” I would suggest this one for the Nrf52840 chip >> https://os.mbed.com/platforms/Nordic-nRF52840-DK/